Hey everyone!
I've been messing around with picotron, and some people keep saying (including myself) that they hope for more buttons to work with or more input methods, but i've discovered something interesting that anyone can use! (until api changes)
I've made a keyboard tester that is fully commented out, and explains how it works and how get_key works and how to use it. to run the cart, click "show" below and copypaste code into picotron, then run it.
I'll explain some things about get_key here.
There are two forms of get_key. get_key_pressed
and get_key_state
. they are very different and are usefull in their own ways.
get_key_pressed(key)
returns true or false, depending on if key key
is pressed. it returns true
for one frame when key key
is pressed.
get_key_state(key)
returns nil or 1, depending on if key key
is HELD. holding the key will make it return 1, and if key is not held, it returns NIL.
as for what to put in key
, there are rules on what a valid keyname is.
"a"
,"space"
and "ctrl"
are all valid keynames.
"A"
,"`"
and "!"
are NOT valid keynames.
Valid keynames include: lowercase letters; numbers; control keys (space,ctrl,backspace,shift,etc)
INVALID keynames include: uppercase letters; symbols; upper key chars; anything you have to press shift for; F keys; programmable hotkeys.
Interestingly enough, "["
and "]"
are also valid keynames, even though no other symbols seem to work.
If you want to use uppercase letters/symbols, you actually arent out of luck! you can write a script to check if shift is held using get_key_state
, then change what keys do what.
I hope this guide/tutorial/cart was useful to you all! just remember that the API still might change before 0.1, so dont expect this to work later down the line. ill try to update this if it stops working, but dont count on it.
I'm almost done with the game!
...that felt really weird to say...
Anyways, the game is almost done, so this will be my last devlog! I'll also keep this one exclusive to the bbs, so I'll make this one count!
Here is everything that I CAN tell you:
Scanning now is how I want it.
Redzones aren't the only thing to worry about...
You'll have at least one ally during the game.
You can't run forever, and wont have to in the end.
Your guiding light costs 2.99 at best buy
Look forward to the release!
If you post anything and go to the "posts" tab in your profile, it always shows at least one comment! (on PC)
I think this is because the number of "comments' shown, really is the number of posts in the thread, and the "thread" starts at your posts "title", and everything below it is a "comment", or a post in the thread.
An easy fix for this, @zep, is instead of displaying the length/how many posts there are in the thread, just change it to be posts_in_thread-1, or whatever your var names are. If it doesn't just work like that, then you can make a new var called something like "num_of_comments_in_thread" and set it to the number of posts in thread minus one, and display that instead of posts in thread.
Ever noticed that when you load an old cart, sometimes it won't load because of API changes? You can see this for yourself if you try loading the popular cart "rougeris". After rougeris's release, 'do' was changed to not work with 'if' and vice versa. On desktop, fixing this is as easy as replacing 'do' with 'then', but on mobile or bbs, not as easy.
Now for the meat of this argument:
Why not make it so that old carts use an older version if pico-8? This doesn't need to be updated for every old cart, but maybe, when you submit a bbs cart from when/if this is changed to an API freeze, the bbs logs what version your cart uses, and when loaded, grabs a specific version of p8, loads it, then runs the cart in it, that would eliminate the possibility of future obsolete carts!
There's really no excuse not to do it, or not to even consider it. Every version of p8 (minus the mysterious "rc") is available to p8 owners on the download page under older versions.
Wait:
FUNCTION WAIT(FRAMES) FOR I=1,FRAMES DO FLIP() END END |
Usage:
Wait(30) pauses cart for 30 frames.
Grab pixel color
FUNCTION GET_PIXEL(X,Y) RETURN PGET(X,Y) END |
Usage:
X=GET_PIXEL(50,40) Gets the color of the pixel at 50,40 and assigns it to X
Get map tile
FUNCTION GET_MTILE(X,Y) RETURN MGET(X/8,Y/8) END |
Returns map tile at x,y
Mini fget mget
FUNCTION GETMFLAG(X,Y) RETURN FGET(MGET(X/8,Y/8)) END |
Returns flag of map tile at x,y
Bit of a shorter one, but I'm half way done with the game! I have around 12 more levels to make, and I'm planning on adding another puzzle mechanic and maybe NPC's.
For now though, I've finally finished the chase mechanics, so I can show those soon. I've also decided calling it "lidar game" is dumb , so for the time being, I am calling it "lights out". Please contact me if you have a better name for it.
I'll have some videos soonish, so look out for that!
I've found some interesting things tucked away in picotron's code, and I'd like to share them!
First off, you can edit and view any file by typing edit name.ext
, where name is the file and ext is the extension.
There are loads of gui features to mess with and ruin, and my favorite is notify_user(<text>)
. When called, it displays "text" in a little notification bar! Even works with vars! Another honorable mention is the gui text editor. It's buggy sometimes, but you can embed a text editor or code editor anywhere you want in your cart!
As for non-gui things, there are many. You can read keyboard presses (get_key_pressed(key)), actively playing sounds, running processes, open files, OPEN files, delete files, change and list directories, all from within your carts! I'm sure there's a way to make a program run in the background, so this could make for custom themes, keyboard remapping, BG music and sound effects, freaking integration with your, YOUR operating system, the list goes on.
Also, in the code for load.lua, you can see that if it finds certain files, it will launch certain editors. This includes .sfx for sounds, .gfx for sprites, .map for... the map, .Lua for code and .pod for who knows what.
Speaking of pods, I feel like I'm the only one exited for them! So much can go in a pod! Images, sprites, sound maybe, open workstations, save data, my net worth, im very exited for wacky old picotron object data
Another really really REALLY cool thing is this: try CDing into /system/util
. Notice anything? Well I did! All those files are the terminal commands! They are also .lua's... (you know where this is going) try this: type "code echo.lua" while your in the util folder to make a new file. At line 1 type print(env().argv[1])
and save it. You should now have "echo.lua" in the util folder. Now type "startup" into the terminal to restart picotron. Now- get ready, type "echo hello!" Into the terminal. That's right. You just wrote your own terminal command! How freaking cool is that!? Incase you wanted to know, how this works is on boot, picotron loads everything in /util/ to be used as a terminal command. env() means "enviornment" and argv[1] means "Argument 1". enviornment is a function that manages ptron's enviornment, and argv is a table that contains any arguments you write in a terminal command! (if you write "load j.p64", j.p64 is the argument).
Last thing, the pinboard. You can actually re-enable it with some work! Just un-comment some code and add some code back, add some of your own, and bam! Because of the way picotron was made, and how easy it is to edit, I'm sure it's not too hard to write a library to put things up there.
The Pixel Toy (TpxT)
2nd picotron game ever made!?
To play, copy and paste code below (click "show") into picotron playground (or actual picotron when it comes out!)
If it says "clipboard can not be read", then don't use base Firefox. if you aren't using base Firefox, then give picotron clipboard permissions in site settings.
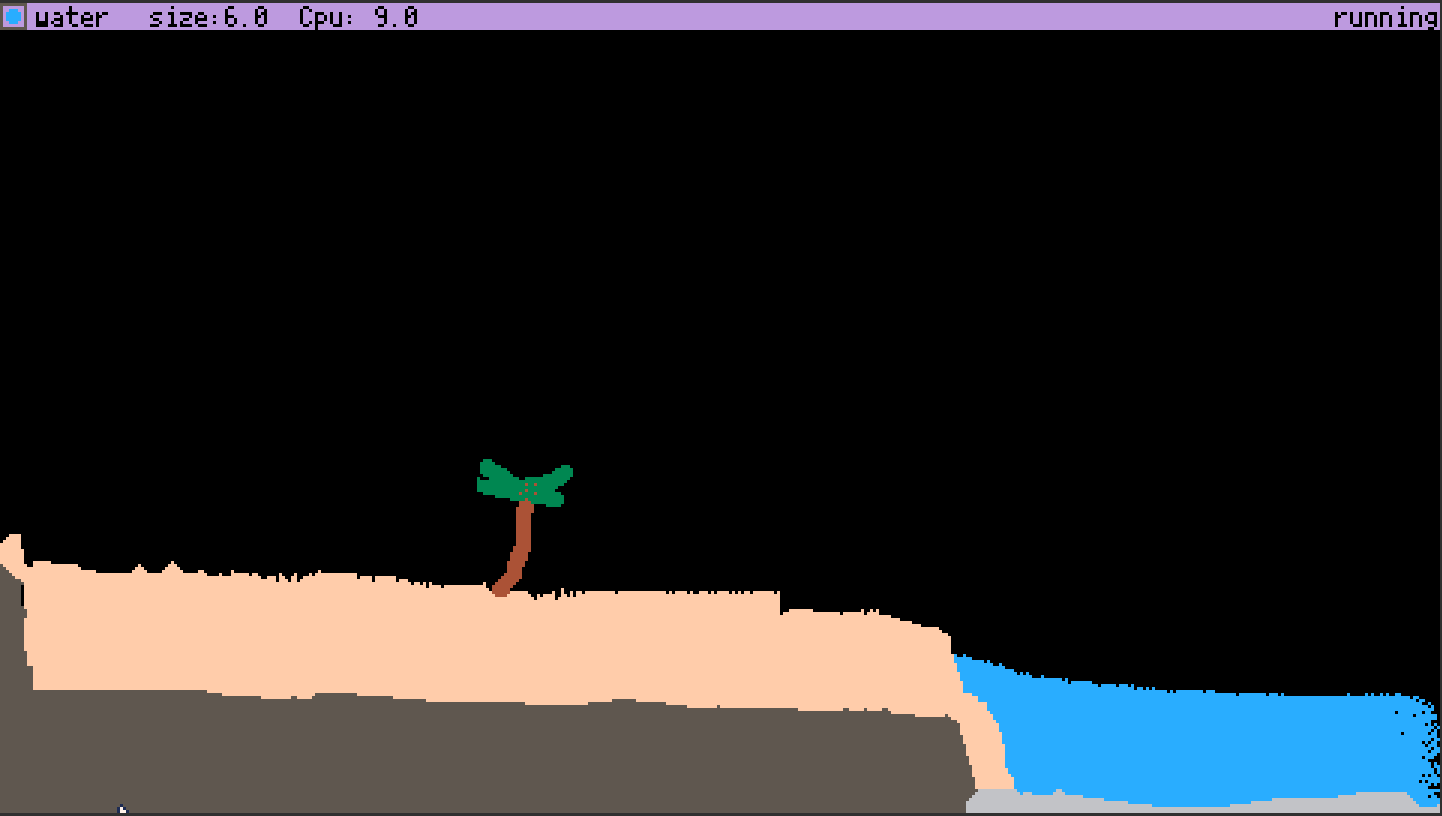
vvv Code vvv
As you can tell, this is based off of The Powder Toy (which you should play!) this is not intended to be a recreation of TPT, more like a demake.
TPT download: https://powdertoy.co.uk/
PicoTron playground: https://www.lexaloffle.com/picotron.php?page=playground
PicoTron main page: (Buy it. gun.png) https://www.picotron.net/
First picotron game ever made: https://www.lexaloffle.com/bbs/?tid=52328
Controls (This game)
Left click - Draw with selected pixel.
Right click - Erase (draw black).
Up/Down - Change brush size.
Left/Right - Change pixel type.
X (hold) - Rectangular fill (Hold x and press and drag Lclick, then release when ready to fill).
Z (hold) - Pauses simulation while held.
Basic reactions (and images!):
Plant and wood burn.
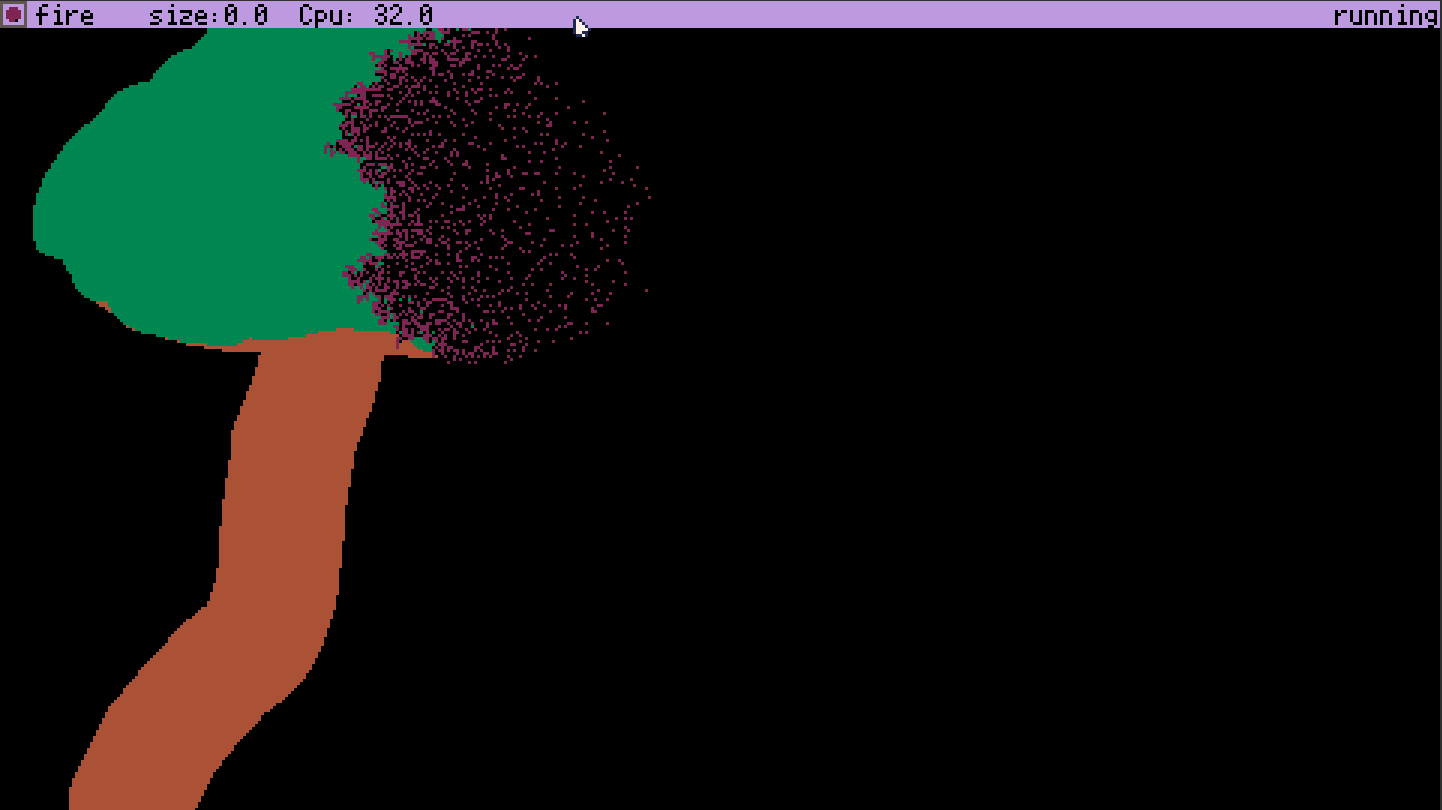
Neutrons eat Deuterium.
Plant eats water.
Water can freeze.
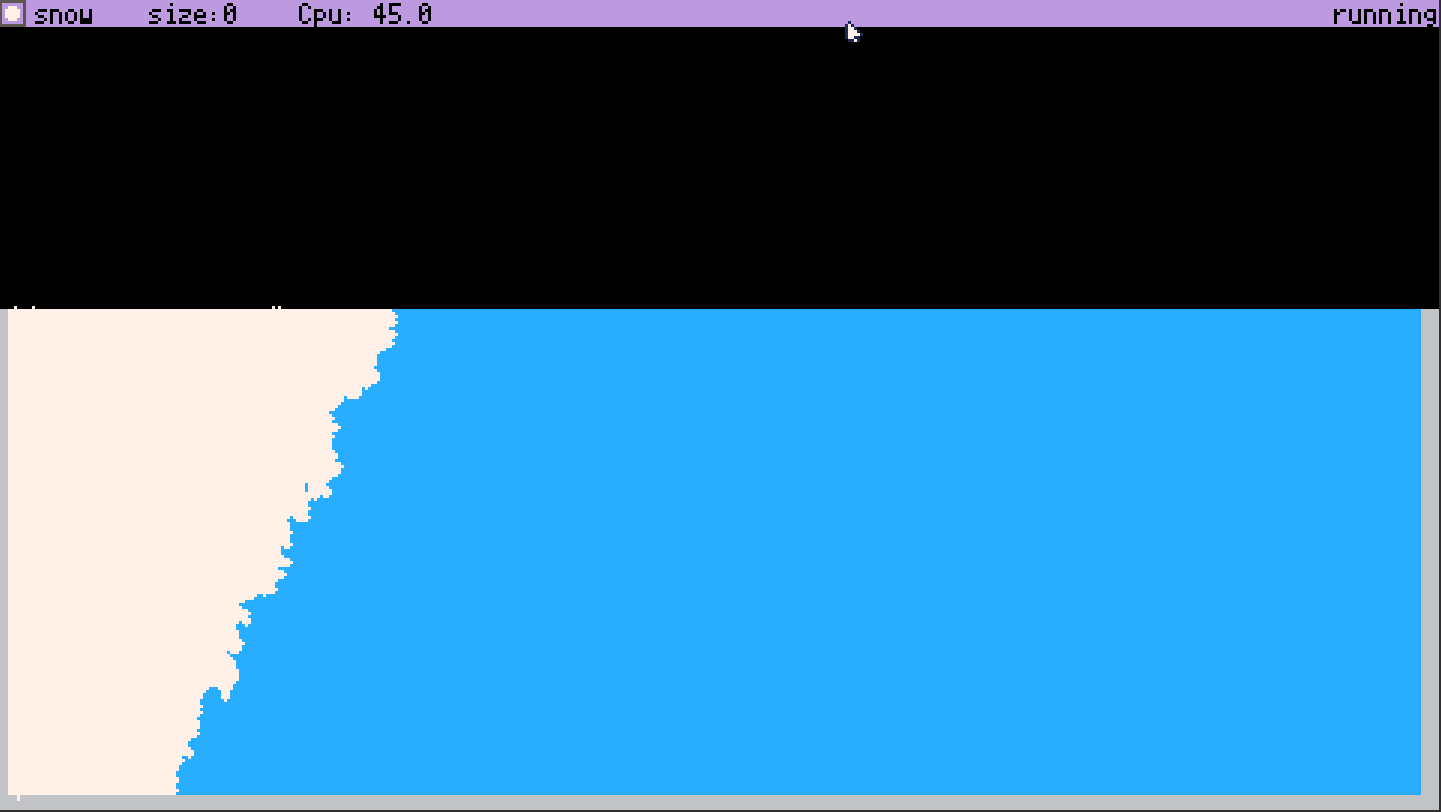
Lava and fire and protons are hot.
Bomb is bomb.
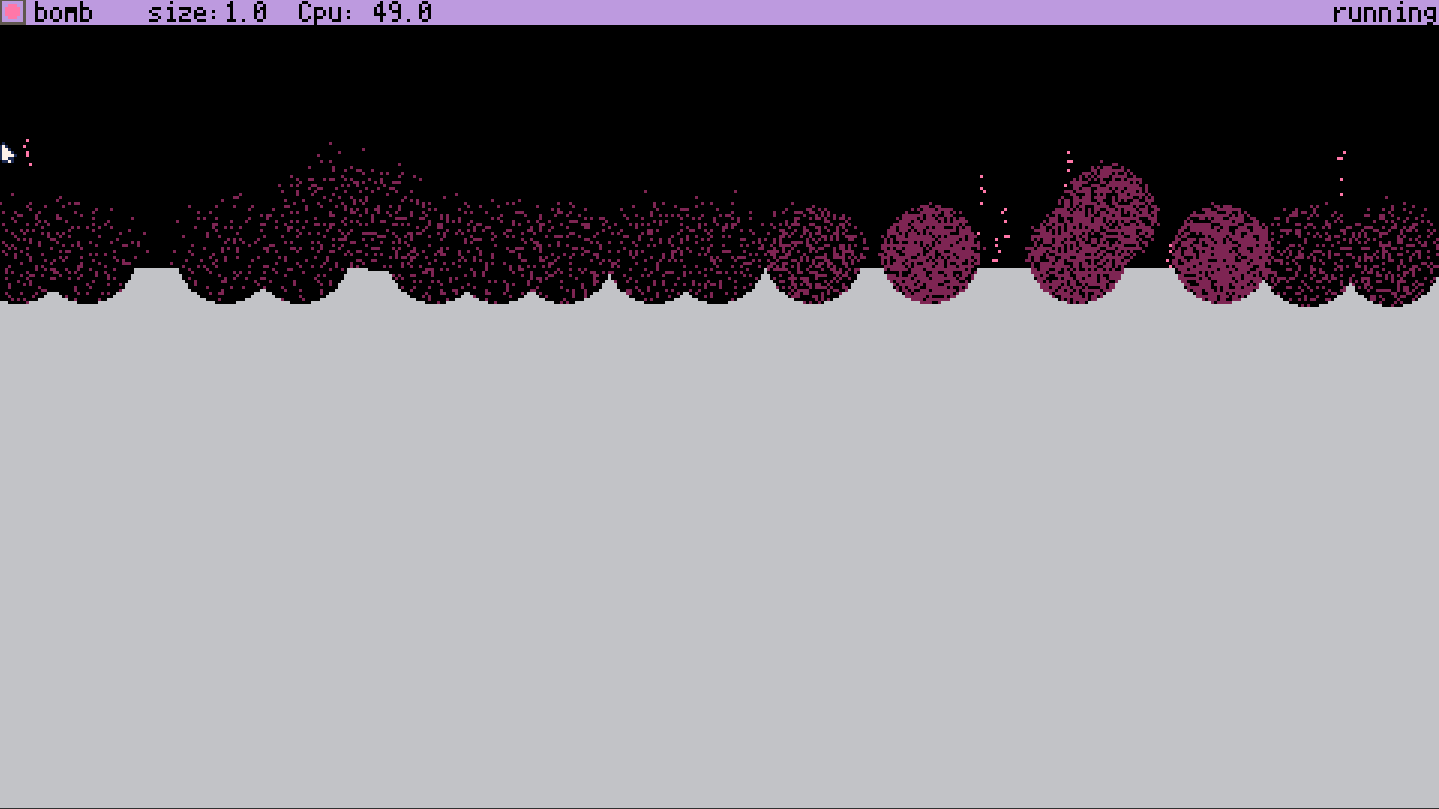
Stone melts when hot.
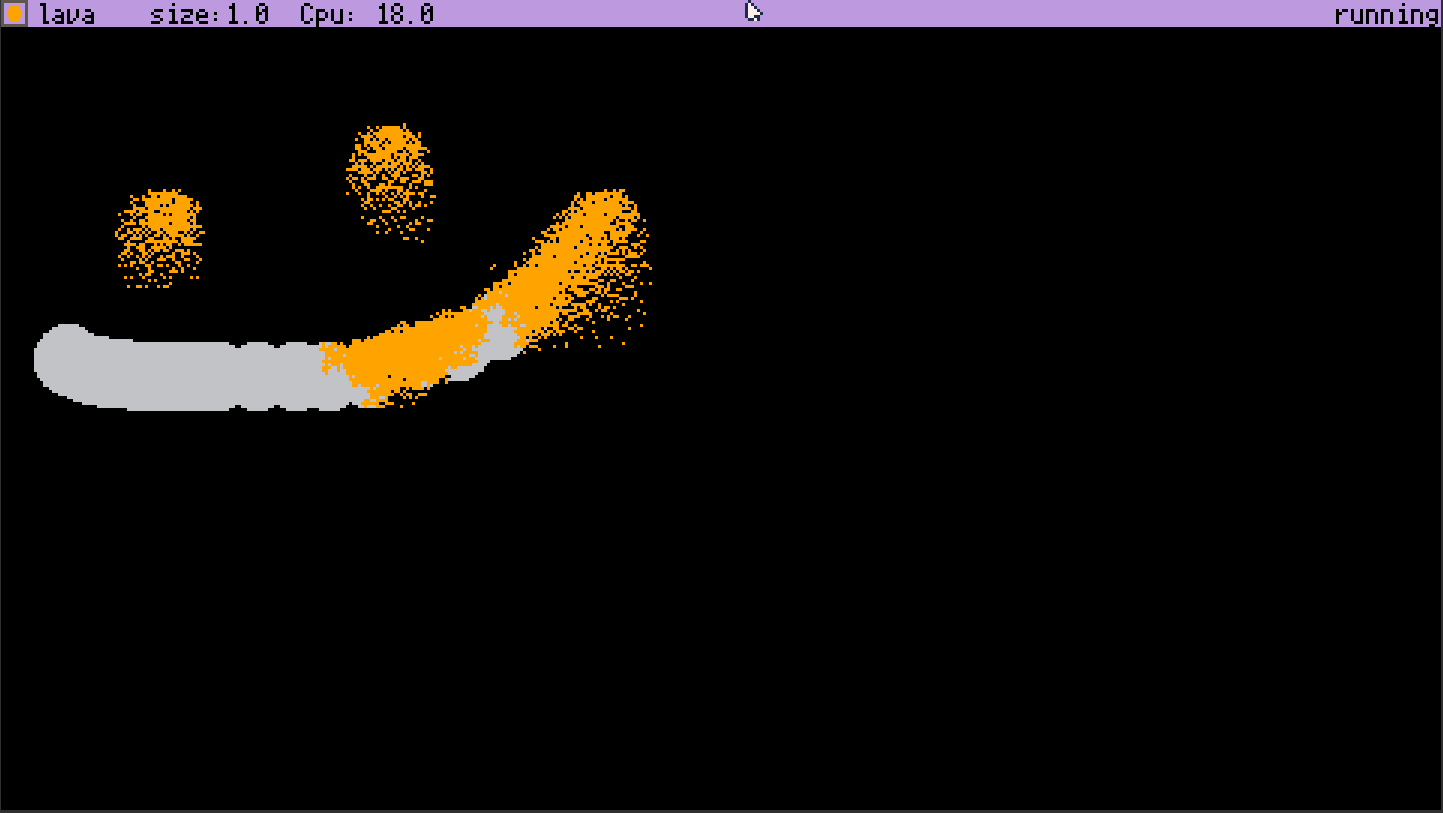
paint does nothing (just for drawing)
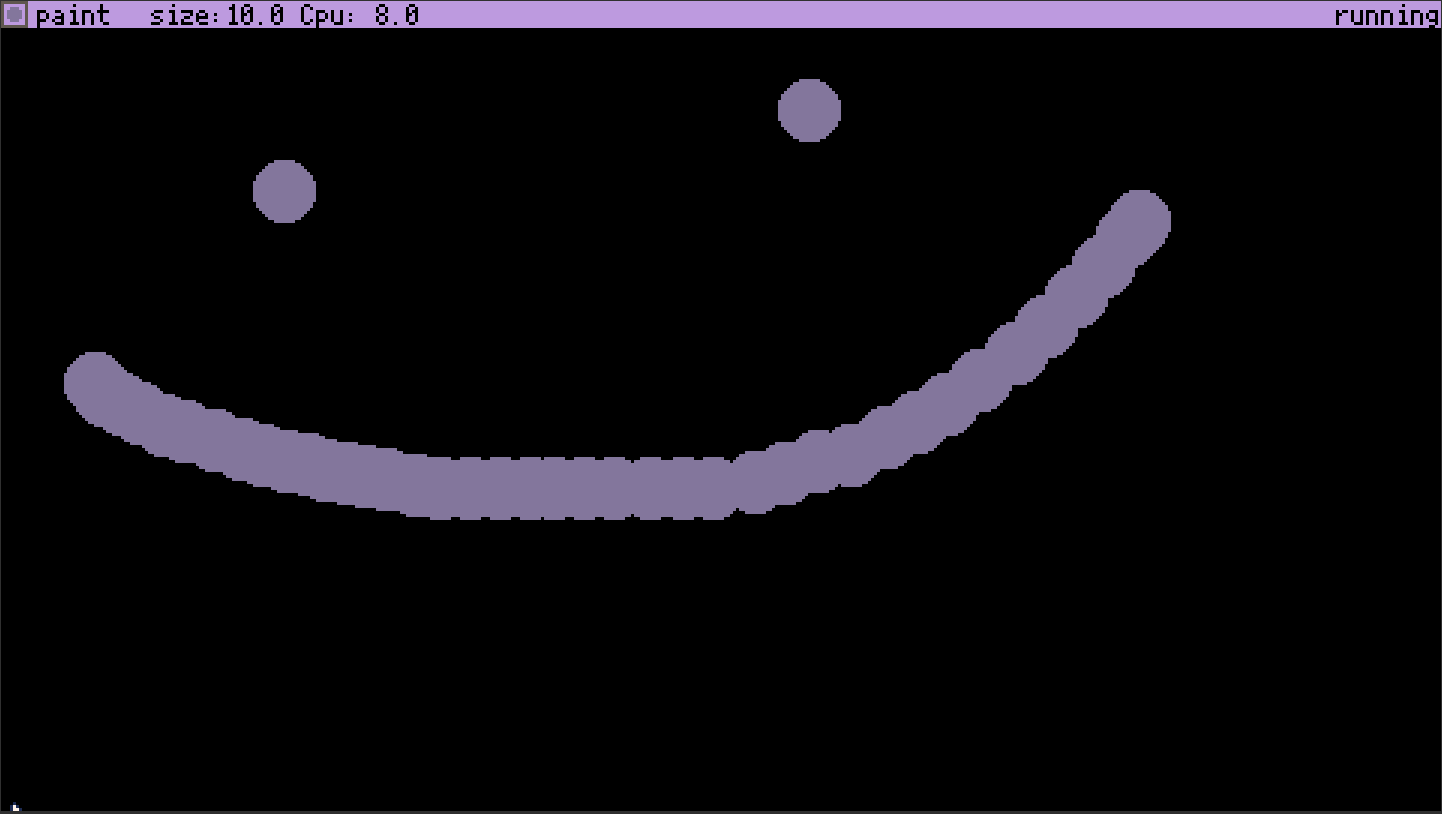
Water and lava dont mix!
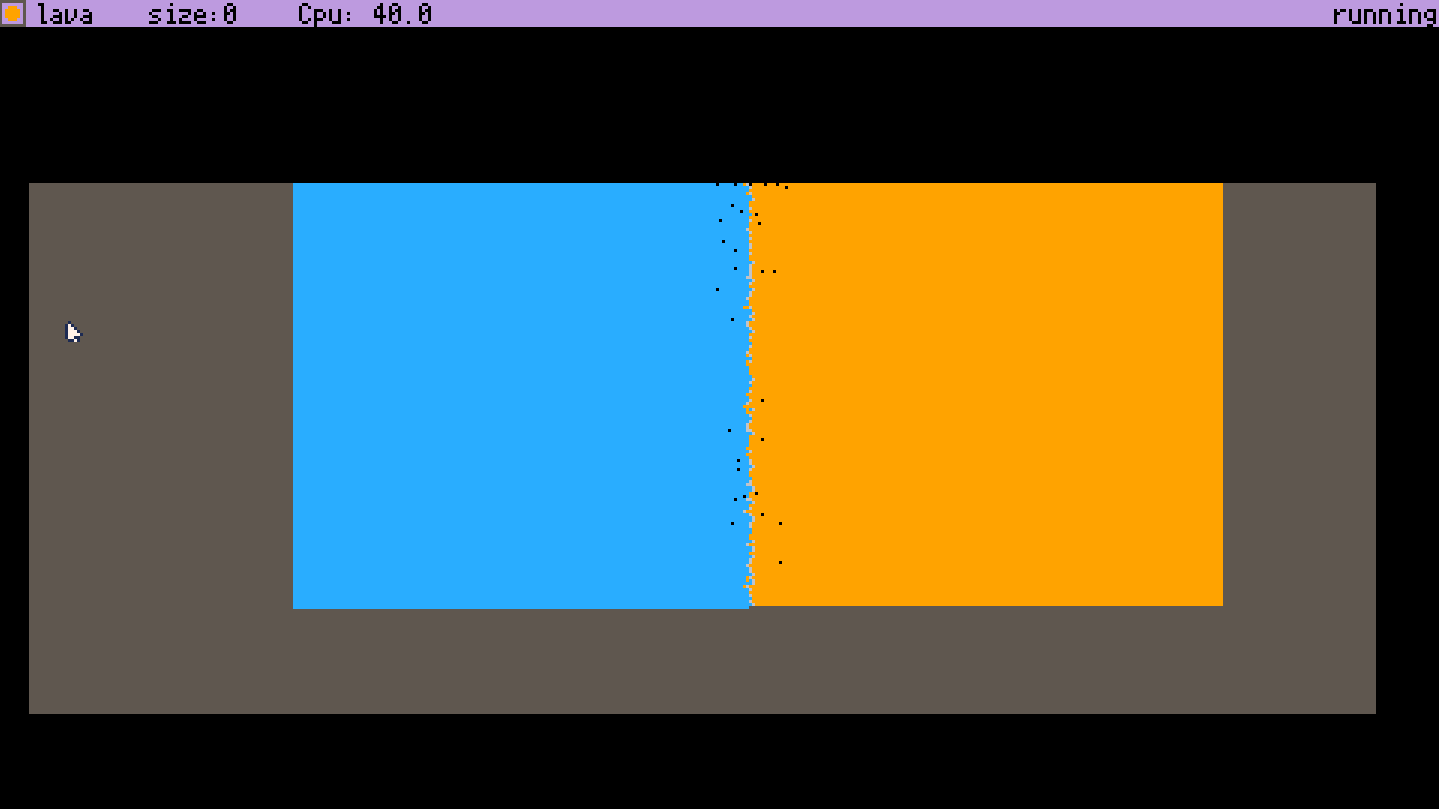
And more?
Comment if you'd like to see any additions! ill add them if they are reasonable/are possible.
Thanks for checking this out! i hope you have fun with it.
1K Demo Collection By @AntiBrain (Me)
This is a collection of 5 democarts that are all under 1KB in total. you can find specific sizes and downloads at the links below or play them at the bottom of this post.
I recommend downloading the executables at my itch page below, because some of them can slow down the web player.
!!EPILEPSY WARNING!!
Many of these carts, especially GradGen and Barf, contain quickly flashing lights and colors. in barfs case, to make as much noise as possible, and in GradGen's case, to create a scrolling effect. if that sounds like it could hurt you, stay away from GradGen and Barf, and show discretion playing any carts here or in any other blog posts. your health always comes first.
Jam:
https://itch.io/jam/pico-1k-2023
Demo Collection Submission Page:
https://itch.io/jam/pico-1k-2023/rate/2262904
Itch.io page w/ downloads:
https://antibrain.itch.io/democollection1k
Individual carts:
GradGen:
DVD:
Circ:
Barf:
Amoeba:
Sizes in compressed bytes are listed on the itch.io page and on the jam submission page. downloads for executables and source code are available on the itch.io page (the antibrain one, not the jam ones)
Cart for PICO-8 1K Jam!
Itch.io link:
Lidar devlog/update #2
Sorry i was gone for so long, but im back now!
Here is another update for my lidar game!
In my last post i promised some puzzle mechanics, so ill reveal them one by one. the first mechanic is in some levels you can find a green tile, that when stepped on it removes red spaces from the map! this can make for some interesting level design where you have to find a switch to progress into a previously blocked-off area. click the link below to see a video showcasing this feature. (also im using twitter for videos because i cant put a .mp4 in a bbs post)
I thought it was strange to restrict update posts to twitter, so here it is!
This is a project ive been working on for a few months and so far its about 1/4th the way done! now what is it though?
This is a game based of Scanner Sombre, a game/trend that was popular for about 2 weeks. Normal scanner sombre is a 1st person horror game where you cannot see and must use a Lidar scanner to sort of "Paint" dots on surfaces to see. after i watched Fundy make his version, i thought to myself: "huh. why cant i do that too?"
Then i decided to make a scanner sombre type game in pico-8! Obviously i do not have the brain power to make a 3D game in pico-8, so i decided to do something more... Creative. I opted to make it a top-down maze game with some puzzle elements and obstacles. The only catch, is that you Cant see the maze. obviously. Puzzle elements i will show later, Ill show the chase sequence after that, and then ill update you all if i add any other big features. for now, here is what i CAN tell you.
The game takes place in a maze where you cannot see anything but pixels you've mapped out, and the player, as well as the HUD. The player is a yellow single pixel and it leaves a trail which deleats quickly. The player trail also removes mapped tiles, so be careful where you step! your main ways of seeing are with X and Z. Pressing X will scan a short area around the player at a slower rate, and pressing Z will shoot a large burst of lines (lazers) that can scan further, but you only have a limited charge and once your charge is used up, it takes a long time to recharge, forcing the player to press on with lower visibility, causing them to make mistakes more often, making the game more challenging. this psudo-horror aspect only really comes into effect during the chase sequence.
Here is a short video showing the first level and some basic mechanics:
I was looking at the Pico-8 changelog and saw that themes were made easier to change. this got me thinking, how hard would it be to make a custom theme, or even accompanying thememaker.exe program? this seemed really cool to me, so i threw myself headfirst into pico-8's files. here are my findings/questions i have.
Firstly, in "pico8.dat" i found that it not only contains data code which i have no idea what it contains, but it also contains a lot of pico-8 code! after a bit of digging, i found that in this order, this pico-8 code is:
1: the pico-8 boot sfx
2:pico-8 democarts
3: i have no clue
I also found around 5 copies of the same lines of terminal syntax (eg 'ls' 'folder' 'load' etc.), which is interesting. i was mainly looking for the color codes that tell pico-8 what colors certain UI elements are, but i could not find them. they had to be in the mess of .dat stuff.
After awhile, i found that pico8.dat is byte-checked, meaning that pico-8 wont boot if pico8.dat has been altered at all. makes sense, it would significantly impact monetary gain if everyone could just write their own pico-8.
after not knowing what to do next, i decided to open pico-8.exe in vscode. i found a lot of terminal command syntax, and licenses for open-source libraries. perfectly normal. i still could not find byte-checks or color codes for UI.
I decided that my next step/idea was to figure out what kind of .dat file pico8.dat was. i saw that it mentions a "POD" file, so thats what i went off of. i could not find anything. i then booted pico-8 and looked in the log.txt. i then saw something interesting. pico-8 seems to be using "CODO" to load files. this "CODO" returned no relevant results online, so i decided just to watch it do its thing. it seems to simply load pico-8, config.txt, the controller thing and also pico8.dat. the most interesting part is that this "CODO" refers to pico8.dat as a "POD" file, which i also cant find anywhere.
If anyone has any ideas of what a "CODO pod file" is, then let me know. if this is scary to you @zep and you dont want pico8 to be decompiled by some sleep-deprived idiot (myself) then ill gladly stop. if not, then custom themes is my next project of anyone wants to help!
Anyone know why the site was down for the past 7ish hours? maintenance? did we get hacked?
EDIT: DOWN AGAIN but it seems were back?
Ive also noticed its impossible to create posts/comments. strange. at least edits work?
@zep do you know whats going on? are we getting ddosed?
it also seems that times are incorrect. i am editing this at 10:09 GMT-7 , and the edit date is wrong.
edit 2: seems posts are back? someone posted abt their movement system, so i guess posts are back. comments still dont work. very odd.
edit 3: i guess posts are gone again. maybe its a me issue. someone try posting.
also ive noticed that if you try to post and cant, changing "pid=" in the search bar to "pid= any number" will, if there is a corresponding post, let you view that post as if you were editing posts. even drafts.
edit 4: ive still been getting update notifications from the automated lexaloffle thing, but the links are wrong. maybe an api is down or something broke somewhere.
edit 5: another short outage? hmm. im beginning to see a pattern.
edit 6: i dont know if this is new, but there is now a "#m" at the end of every lexaloffle url. huh.
edit 7: i guess now its just for some pages.
test
edit 8: seems like some people can still post/comment. i dont know why. maybe @zep does. i dont know if its daytime in japan (or wherever zep/the website developer is) right now, or if they are even awake, but my guess is it'll hopefully be fixed tomorrow. i have to go to sleep because its midnight where i am. gn everyone. lets hope its not serious and its simply server shenanigans and not a targeted attack.
Mangle08 (!!!SEIZURE WARNING!!!)
What this cart does is poke as many values as possible to mangle pico-8 into an almost unusable state. it will mess with colors, drawing, the map(?), and sounds.
This cart is best experienced when downloaded and ran on desktop pico-8, or at https://www.pico-8-edu.com/ . just type "load #mangle" to load it. after running it for a bit try messing around with the sprite editor, sounds, map, or anything else.
GROW
Simple Democart i made.
I came across this idea when working on my other game (info on my twitter :) ), and decided to horribly code unglue it from that game and edit it into a little pico-8 democart! I tried to keep it similar to p8's builtin demos, but i couldn't help myself from adding little sounds and stuff. (Also if you want it @zep , its yours :) ) feel free to yoink code from this cart if you feel like it! i commented out everything that needs it so that even beginners can make sense of whats going on under the floorboards. er- what makes the-- never mind. i also left some map spaces open if you want to make your own levels!
Thank you all from the pico-8 community for not yelling about my awful code! i really appreciate all the support from my other projects and im glad im apart of this little community.
Thank you so much for checking this out! have fun!
Random Cart loader
This cartridge loads a random numerical id cartridge of bbs. basically any cart on bbs thats cart id is a number.
Update 2.0 : Compress code + fix display bug
PICOTEMPLATE
Nothing special, nothing crazy. just a simple template cart for making games.
How to use:
click the little cartridge icon in the corner of the cart and right click the image to download. then just load it and start making your own cart! don't forget to save it as a new filename after you make something so that it doesn't save to the template cart.
KAIZOLESTE: A NEW MOUNTAIN
A new mountain has been discovered where the impossible becomes possible and chaos becomes order and order becomes chaos. Random peaks, Deep caves, and penguins galore, Madeline faces her hardest challenge yet in Kaizoleste.
IMPORTANT:
This mod has SAAAAAVE DAAATAAA!!!! all of your progress is saved no matter where you play, even online! all your data is saved including: your current level; your total time played; your death count and; your berry count! if you click "delete save" in the pause menu, all of your savedata is gone. if you accidently click it, no worries! just beat your current level and you wont lose any progress.
Also credits to evercore team for evercore, because i used it as a base for this mod.
notes:
This mod REQUIRES spike clips and spike jumps to beat. jemskip is NOT possible to my knowledge, and all levels and berries are possible, but some require harder tricks. helpful arrow signs indicate how to perform certain tricks, but they go away later on. For more info on speedrunning tricks and tech, goto https://celesteclassic.github.io/glossary/ !
This was my absolute favorite thing in general to make. the journey of creating this mod took about 1 year and every minute was worth it. thank you so much for playing and cheering me on and i hope you enjoy this mod as much as i enjoyed making it
Heres my best time:
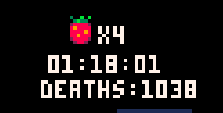
Congrats to @Catastrophic Cal for beating my time!
READ THIS FIRST
Click the cartridge icon at the bottom of the cart and right click on the image to save it, then just plop it in your /carts/
folder. To use it in desktop pico-8, just put it in your carts folder. For use in pico-8-edu, go to immediate mode (the terminal) and type load #picobrowser
, then type save [NAME].p8
where name is whatever you want. then, in pico-8 or edu, just type load name.p8
, again where [name] is what you named the file.
more info:
This cart was made using @dw817 's "free roaming directory" cart. Their version, however could only see in /carts/ and couldn't load anything.
Features:
. Displays a real visualization of all your files in your carts folder.
. Lets you go in and out of folders in /carts/
. Lets you load .p8 and .p8.png files.
. Lets you load BBS carts ( more on that below :) )
. Lets you return to the browser within loaded cart using a breadcrumb system
. Can load any .p8 or .p8.png file you throw at it (if you don't mess with the file extension)
. Has the best sound effects in the entire world (big claim, I know)
. Scroll bar so you don't get lost
. Only has 1 unfixable bug! ( integer limit :p )
Changelog:
v3.0
v3.1
v3.2
v3.3
v3.31
v3.32
v3.33
v3.4
v4.0
v5.0
Todo:
Important:
This cart can load BBS carts from your carts folder! Simply press 🅾️ and enter your cartridge's bbs ID, Without the hashtag/pound symbol (#).
the only bug I cant fix right now is integer limits. if you have more then 30k+ files then... well you need to figure something out man, and also the cart will break. if you have a really long folder path that's more then the string limit deep, then you wont be able to go any deeper. so basically: don't have too many files and don't have too many subdirectories.
Thanks to @dw817 for the original cart.
IMPORTANT 2: I just realized that a folder in the cart preview is named "joke". That does not mean this cart is a joke. I just needed to make folders for the cart label and just wrote the first 3 things that I thought of.
final notes:
I had a lot of fun making this! its the best that it could be as of posting this, and i really like how it came out. i did end up adding some useless features that really pull everything together and im proud of that. my vision with this cart is that maybe someday it will be the go-to way to browse files from within pico-8's os. no more annoying ls and cd commands and finally a nice p8 style UI. maybe one day it will be a built-in pico-8 feature. ( @zep :O ) in all, this was my favorite cart to make so far and i hope you all enjoy it as much as i enjoyed making it.
Final notes 2 (I am an idiot)
I didnt know splore had a file browser in it, so i guess this software is completely redundant on desktop pico-8, but it is still useful on devices that dont have splore! (like edu!)
Thanks for taking the time to read my documentary on my silly file browser!
ALSO, As of v4.0, with the addition of mouse control, maybe it is better? let me know if you think so!
View Older Posts